Start a Django project
To start a Django project you
must use the django-admin
executable that comes with Django. After
you install Django, this executable should be
accessible from any directory on your system (e.g. installed under
/usr/bin/
,/usr/local/bin/
or the
/bin/
directory of a virtualenv).
The django-admin
offers various subcommands you'll use extensively for your daily
work with Django projects. But it's the startproject
subcommand you'll use first, since it creates the initial structure
of a Django project. The startproject
subcommand
receives a single argument to indicate the name of project, as
illustrated in listing 1-14.
Listing 1-14. Django django-admin startproject
command
# Create a project called coffeehouse [user@~]$ django-admin startproject coffeehouse # Create a project called sportstats [user@~]$ django-admin startproject sportstats
A Django project name must adhere
to Python's identifier[9] naming conventions, which are:
identifier ::= (letter|"_") (letter | digit | "_")*
.
In layman's terms, a Django project name must start with a letter or underscore, followed by a sequence of numbers, letters or underscores. Therefore, numbers aren't allowed as the first character of a Django project name. In addition, special characters and spaces also aren't allowed anywhere in a Django project name. This adherence to Python's identifier naming conventions, is due to a Django project name serving to structure directories and Python package names.
Upon executing django-admin
startproject <project_name>
, a directory called
<project_name>
is created containing the default
Django project structure. The default Django project structure is
illustrated in listing 1-15.
Listing 1-15. Django project structure
+<BASE_DIR_project_name> | +----manage.py | +---+-<PROJECT_DIR_project_name> | +-asgi.py +-__init__.py +-settings.py +-urls.py +-wsgi.py
If you inspect the directory
layout, you'll notice there are two directories with the
<project_name>
value. The top
level Django project directory is referred to as the BASE_DIR
, which
includes the manage.py
file and the other
sub-directory based on the project name. I will refer to the
second level sub-directory -- which includes the
asgi.py
, __init__.py
, settings.py
,
urls.py
and wsgi.py
files -- as the PROJECT_DIR
. Next, I'll describe the purpose of each
file in listing 1-15.
manage.py
.- Runs project specific tasks. Just asdjango-admin
is used to execute system wide Django tasks,manage.py
is used to execute project specific tasks.asgi.py
.- Contains ASGI configuration properties for the Django project. ASGI is the recommended approach to deploy Django applications asynchronously on production (i.e. to the public). You don't need to setup ASGI to develop Django applications.__init__.py
.- Python file that allows Python packages to be imported from directories where it's present. Note__init__.py
is not Django specific, it's a generic file used in almost all Python applications.settings.py
.- Contains the configuration settings for the Django project.urls.py
.- Contains URL patterns for the Django project.wsgi.py
.- Contains WSGI configuration properties for the Django project. WSGI is the recommended approach to deploy Django applications on production (i.e. to the public). You don't need to setup WSGI to develop Django applications.
Tip Rename a project'sBASE_DIR
. Having two nested directories with the same name in a Django project can lead to confusion, especially if you deal with Python package import issues. To save yourself trouble, I recommend you rename theBASE_DIR
to something different than the project name (e.g. rename, capitalize or shorten the name to make it different than thePROJECT_DIR
).
Caution Do not rename thePROJECT_DIR
. ThePROJECT_DIR
name is hard-coded into some project files (e.g.settings.py
,asgi.py
andwsgi.py
) so do not change its name. If you need to rename thePROJECT_DIR
it's simpler to create another project with a new name.
Now that you're familiar with the
default Django project structure, let's see the default Django
project in a browser. All Django projects have a built-in web
server to observe an application in a browser as changes are made
to project files. Placed in the BASE_DIR
of a Django
project -- where the manage.py
file is -- run the
command python manage.py runserver
as shown in listing
1-16.
Listing 1-16. Start Django development web server provided by manage.py
[user@coffeehouse ~]$ python manage.py runserver Watching for file changes with StatReloader Performing system checks... System check identified no issues (0 silenced). You have 18 unapplied migration(s). Your project may not work properly until you apply the migrations for app(s): admin, auth, contenttypes, sessions. Run 'python manage.py migrate' to apply them. February 05, 2022 - 03:32:47 Django version 4.0.2, using settings 'coffeehouse.settings' Starting development server at http://127.0.0.1:8000/ Quit the server with CONTROL-C.
As illustrated in listing 1-16,
the command python manage.py runserver
starts a
development web server on http://127.0.0.1:8000/ -- which
is the local address on your system. Don't worry about the
'unapplied migration(s)'
message for the moment, I'll
address it in the upcoming section on setting up a database for a
Django project. Next, if you open a browser and point it to the
address http://127.0.0.1:8000/ you should see the default
home page for a Django project illustrated in figure 1-3.
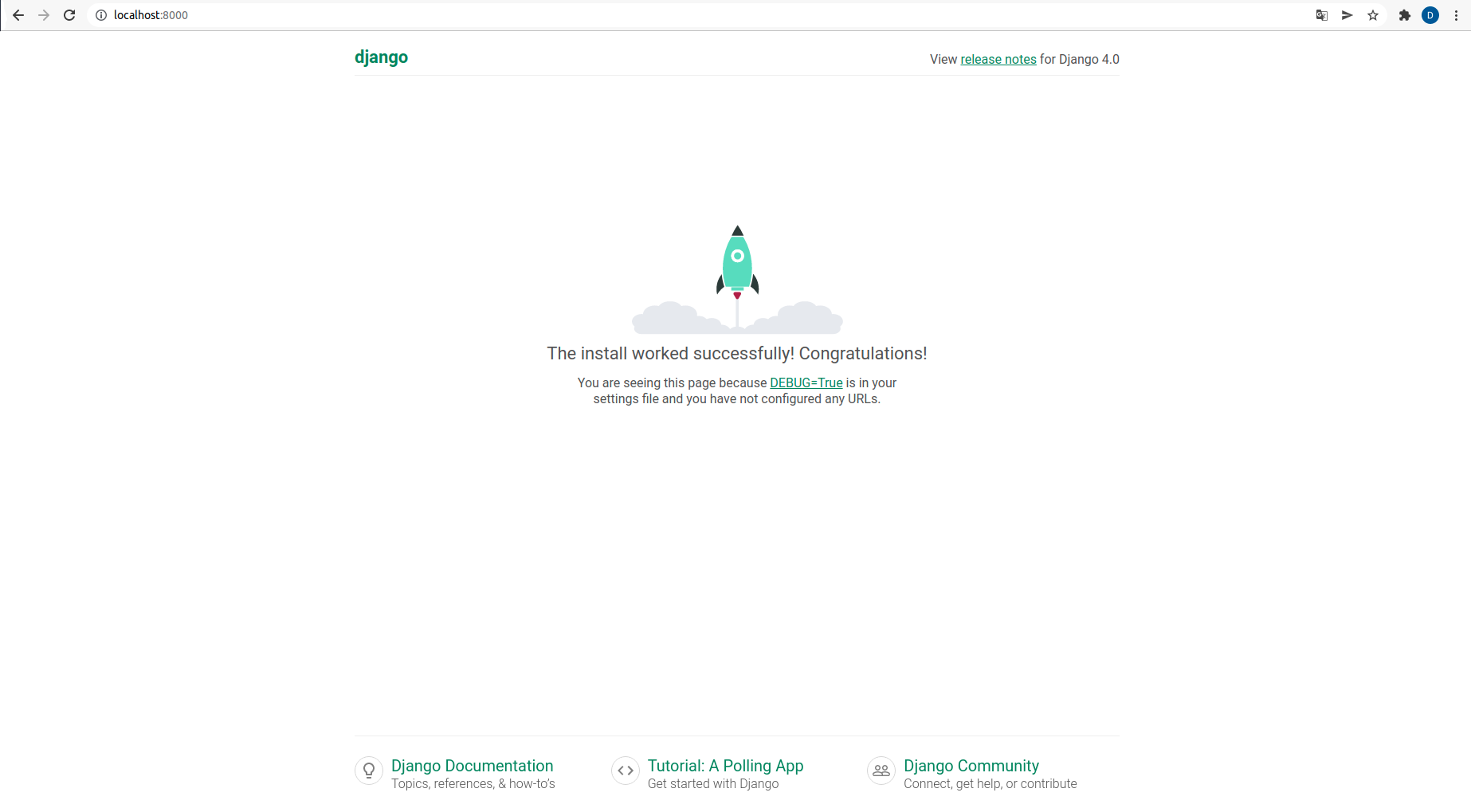
Figure 1-3. Default home page for a Django project
Sometimes it's convenient to
alter the default address and port for Django's development web
server. This can be due to the default port being busy by another
service or the need to bind the web server to a non-local address
so someone on a remote machine can view the development server.
This is easily achieved by appending either the port or full
address:port string to the python manage.py runserver
command, as shown in the various examples in listing 1-17.
Listing 1-17. Start Django development web server on different address and port
# Run the development server on the local address and port 4345 (http://127.0.0.1:4345/) python manage.py runserver 4345 # Run the dev server on the 96.126.104.88 address and port 80 (http://96.126.104.88/) python manage.py runserver 96.126.104.88:80 # Run the dev server on the 192.168.0.2 address and port 8888 (http://192.168.0.2:8888/) python manage.py runserver 192.168.0.2:8888